vscode - Extension Ripping for Dockerfile builds
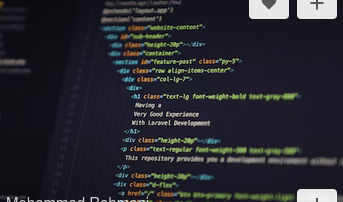
Here is some simple code that will read your vscode.json file and rewrite it in a format that can be used in a Docker build (Dockerfile):
You will need this added to your Dockerfile so that it can install vscode extensions headless + the boiler plate code to install vs code.
Before you build if you get 'max depth exceeded' you may need to clean your images:
docker rmi -f $(docker images -a -q)
RUN DEBIAN_FRONTEND=noninteractive dpkg -i /files/vscode.deb
RUN DEBIAN_FRONTEND=noninteractive apt-get install -y rsync curl
RUN DEBIAN_FRONTEND=noninteractive curl -fsSL https://code-server.dev/install.sh | sh
Once you have these added then this python script will 'rip-n-write' your extension installs.
import re
# Press Shift+F10 to execute it or replace it with your code.
# Press Double Shift to search everywhere for classes, files, tool windows, actions, and settings.
with open('vscode.json', 'r') as g:
mylines = g.readlines()
total_matches = ''
matches = re.findall('"id":"[^"]+"', mylines[0])
ccount = 0
for match in matches:
if '.' in match:
match = match.replace('"id":"', '')
while '"' in match:
match = match.replace('"', '')
ccount += 1
total_matches += match + ' '
if ccount >= 8:
print(f"RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension {total_matches}")
ccount = 0
total_matches = ''
It will produce compounding install statements wrapping at every 8. This is important as Dockerfile build depth's can break once your Dockerfile passes 250..
RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension ms-python.python ms-python.vscode-pylance ms-vscode-remote.remote-containers ms-azuretools.vscode-docker vscodevim.vim eamodio.gitlens mtxr.sqltools redhat.vscode-yaml
RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension ms-kubernetes-tools.vscode-kubernetes-tools googlecloudtools.cloudcode kevinrose.vsc-python-indent njpwerner.autodocstring wholroyd.jinja batisteo.vscode-django visualstudioexptteam.intellicode-api-usage-examples donjayamanne.python-environment-manager
RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension tht13.python visualstudioexptteam.vscodeintellicode donjayamanne.python-extension-pack tushortz.python-extended-snippets mgesbert.python-path ms-vscode.test-adapter-converter seanwu.vscode-qt-for-python hbenl.vscode-test-explorer
RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension littlefoxteam.vscode-python-test-adapter 076923.python-image-preview njqdev.vscode-python-typehint cstrap.python-snippets ms-vscode.cpptools benjamin-simmonds.pythoncpp-debug kaih2o.python-resource-monitor mukundan.python-docs
RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension xirider.livecode geecode.geecode-python magicstack.magicpython danields761.dracula-theme-from-intellij-pythoned yzhang.markdown-all-in-one davidanson.vscode-markdownlint nils-ballmann.python-coding-tools editorconfig.editorconfig
RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension wakatime.vscode-wakatime aaron-bond.better-comments magicstack.chromodynamics shan.code-settings-sync coenraads.bracket-pair-colorizer-2 emeraldwalk.runonsave esbenp.prettier-vscode formulahendry.code-runner
RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension streetsidesoftware.code-spell-checker spywhere.guides almenon.arepl ms-vsliveshare.vsliveshare fabioz.vscode-pydev ms-pyright.pyright hbenl.vscode-test-explorer-liveshare jillmnolan.python-essentials
RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension ms-toolsai.jupyter-keymap ms-toolsai.vscode-jupyter-slideshow ms-toolsai.vscode-jupyter-cell-tags ms-toolsai.jupyter-renderers ms-toolsai.jupyter ericsia.pythonsnippets3 leojhonsong.python-extension-pack rickywhite.python-template-snippets
RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension graykode.ai-docstring ahadcove.python-quick-print ptweir.python-string-sql hyesun.py-paste-indent akhail.darcula-theme-python swellaby.python-pack filwaline.vscode-postfix-python patrick91.python-dependencies-vscode
RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension alexrsagen.python-debug-address-prompt ms-vscode.anycode-python eliazbobadilla.python-ultisnippets austin.code-gnu-global ms-vscode.cpptools-themes ms-vscode.cmake-tools jeff-hykin.better-cpp-syntax twxs.cmake
RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension ms-vscode.cpptools-extension-pack vadimcn.vscode-lldb danielpinto8zz6.c-cpp-compile-run franneck94.c-cpp-runner mitaki28.vscode-clang kaysonwu.cpptask jbenden.c-cpp-flylint danielpinto8zz6.c-cpp-project-generator
RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension acharluk.easy-cpp-projects davidbroetje.algorithm-mnemonics-vscode vscjava.vscode-java-debug vscjava.vscode-java-dependency vscjava.vscode-maven vscjava.vscode-spring-initializr redhat.java donjayamanne.javadebugger
RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension vscjava.vscode-gradle vscjava.vscode-java-test youmaycallmev.vscode-java-saber vscjava.vscode-java-pack dsnake.java-debug georgewfraser.vscode-javac shengchen.vscode-checkstyle caolin.java-run
RUN DEBIAN_FRONTEND=noninteractive code-server --install-extension sohibe.java-generate-setters-getters xabikos.javascriptsnippets ithildir.java-properties dgileadi.java-decompiler ms-vscode.vscode-typescript-next walkme.java-extension-pack tushortz.java-imports-snippets tushortz.java-snippets
To push to repository:
docker tag image <docker_user>/image
docker push <docker_user>/image