http: python: Understanding the Cookie Cycle.
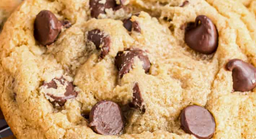
Cookies can be quite confusing. Hopefully this will help you understand it.
How do http sessions actually work? Here is a programmers break down for back-end developers and will give you hopefully a comprehensive understanding:
Consider the following python code block:
import socketserver
from http.server import *
from http import cookies
class Shandler(BaseHTTPRequestHandler):
def __init__(self, request: bytes, client_address: tuple[str, int], server: socketserver.BaseServer):
super().__init__(request, client_address, server)
self.cookie = None
def do_GET(self):
self.cookie = self.headers.get("Cookie")
if self.cookie == None:
print(f"Setting cookie now")
self.send_response(200)
self.send_header("Set-Cookie", "mycookie=213a238d8; Max-Age=50000")
self.end_headers()
return("New Connection - setting cookie")
else:
print(f"Cookie already set: {self.cookie}")
return("Cookie already set!")
address = ('', 8001)
handler = Shandler
server = HTTPServer(address, handler)
while True:
server.serve_forever()
- do_GET is a GENERIC class of BashHTTPRequestHandler when the browser posts a 'GET'
- self.headers.get("Cookie") will fetch the http header cookies from the tuple object inside the current session. The first time there is no cookie so it is None.
- Think parallel. This code would exist - and run in parallel as different session users access it. Thus it would have a completely different set of cookie values if another browser session were to access it.
Setting the cookie on first time:
if self.cookie == None:
print(f"Setting cookie now")
self.send_response(200)
self.send_header("Set-Cookie", "mycookie=213a238d8; Max-Age=50000")
self.end_headers()
- 'Set-Cookie' is a standard http transport header accept by industry standard now. Here
- 'Set-Cookie' effectively 'seeds' the browser with the cookie the server requires it to retain for the duration of its session or for Max-Age. If no duration is specified it is set for 'Session' which means when the user closes the browser the cookie disappears.
The first time this is called we get:

After the browser is seeded with the cookie each time it pulls the same site it will pass this cookie back.
On the second poll of the site we then get the result back to the server which can confirm it is the same user - as in:
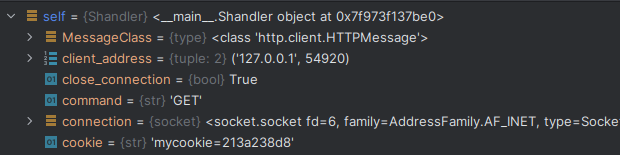
This is as simply as it can be explained hopefully and can get the developing programming past the cookie hurdle!
Understand Granularity:
- What many developers would not necessarily note is this BaseHTTPServer works at the IP level. What this means is if two people behind a NAT firewall accessed the same site the server would see the same cookies regardless of the client port. It is up to the programmer to add in the granularity for the current client port / client user. Thus users using VPN's could possibly see the cookie session of another user in a incorrectly configured program! Usually production level systems handle for this but if you are writing for this - it is up to you to program in the correct logic to handle this. The base way to to compare the incoming cookie to the existing table.
- Simplifying this in the above example the browser happened to be holding the cookies from a phpsqladmin session and actually passed them because the browser saw the same IP / port numbers from that session and this code block actually intercepted a different sessions cookies! Therefore not only must the server look for a cookie - it must look for the 'right' cookie that matches it's logic.